Table of contents
ASP.NET Core and C#: The Backbone
In 2021 Umbraco switched to .NET Core, as I wrote here: The Cross-Platform Revolution: Umbraco and .NET Core.
You must learn ASP .NET Core & C# to build Umbraco applications.
It's like peanut butter and jelly – a perfect match.
Learn more about C#
Start working with C# by checking out the official C# documentation.
It's filled with easy-to-follow guides and excellent examples that will make learning C# more fun and less of a headache.
Learn more about ASP .NET Core
ASP.NET Core is your gateway to building responsive and dynamic web applications.
To start mastering it, check out the official ASP.NET Core documentation.
It's your ultimate guidebook, offering everything from basic tutorials to advanced features.
You’ll find step-by-step instructions, best practices, and practical tips to harness the full potential of ASP.NET Core in your Umbraco development journey.
Discover the Best of ASP.NET Core
To get the full scoop on what ASP.NET Core offers, check out our detailed article, "ASP.NET Core Essential Features."
Here, you'll find a deep dive into the features that make ASP.NET Core stand out in the world of web development.
Defensive Programming: Ensuring Robust Umbraco Applications
The importance of defensive programming cannot be overstated in web development, particularly with complex platforms like Umbraco.
Defensive programming is a proactive approach that anticipates potential failures in a program by writing preventive code to handle these situations gracefully.
Why Defensive Programming Matters
Defensive programming is crucial because it helps maintain an application's integrity and reliability under unexpected conditions.
It involves validating input, implementing fail-safe measures, and ensuring consistent outputs, which are essential for securing web applications against malicious attacks or unintended misuse.
Key Principles of Defensive Programming
- Input Validation: Always validate user input to prevent injections and other malicious attacks.
- Fail-Safe Defaults: Set safe defaults in case of system failures, ensuring the system remains secure.
- Consistent Error Handling: Use consistent error-handling practices to prevent crashes and data corruption.
- Logging and Monitoring: Implement comprehensive logging to track unexpected behaviors and potential breaches.
Further Learning and Examples
To learn more about implementing defensive programming in your Umbraco projects, our comprehensive guide, Top Defensive Programming Principles with Examples, offers practical advice and detailed examples that you can apply directly to your development practices.
This guide will help you understand the theoretical underpinnings and provide real-world applications and coding samples.
By integrating these defensive programming techniques into your development workflow, you can significantly enhance the security and resilience of your Umbraco applications, ensuring they perform reliably and securely, even under adverse conditions.
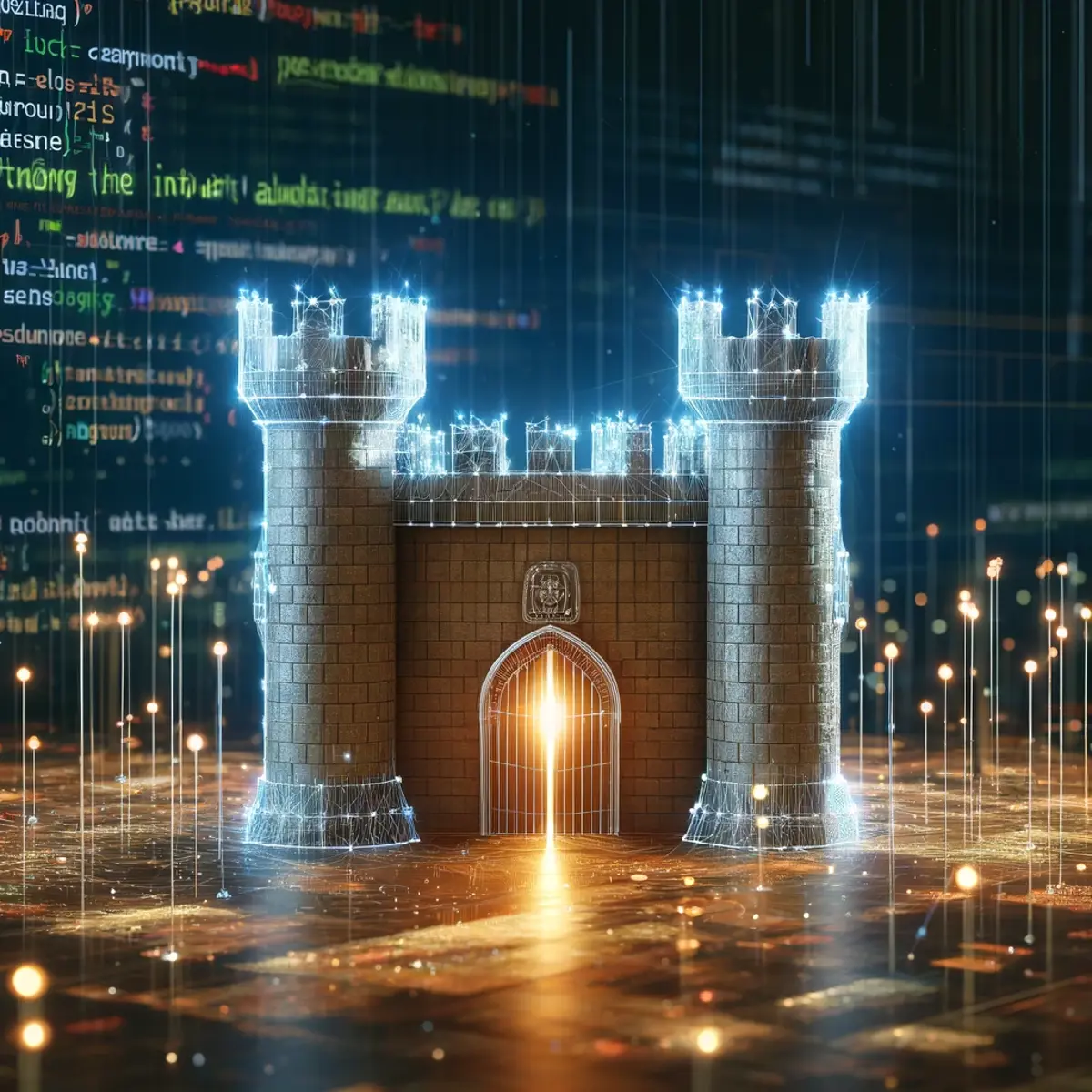
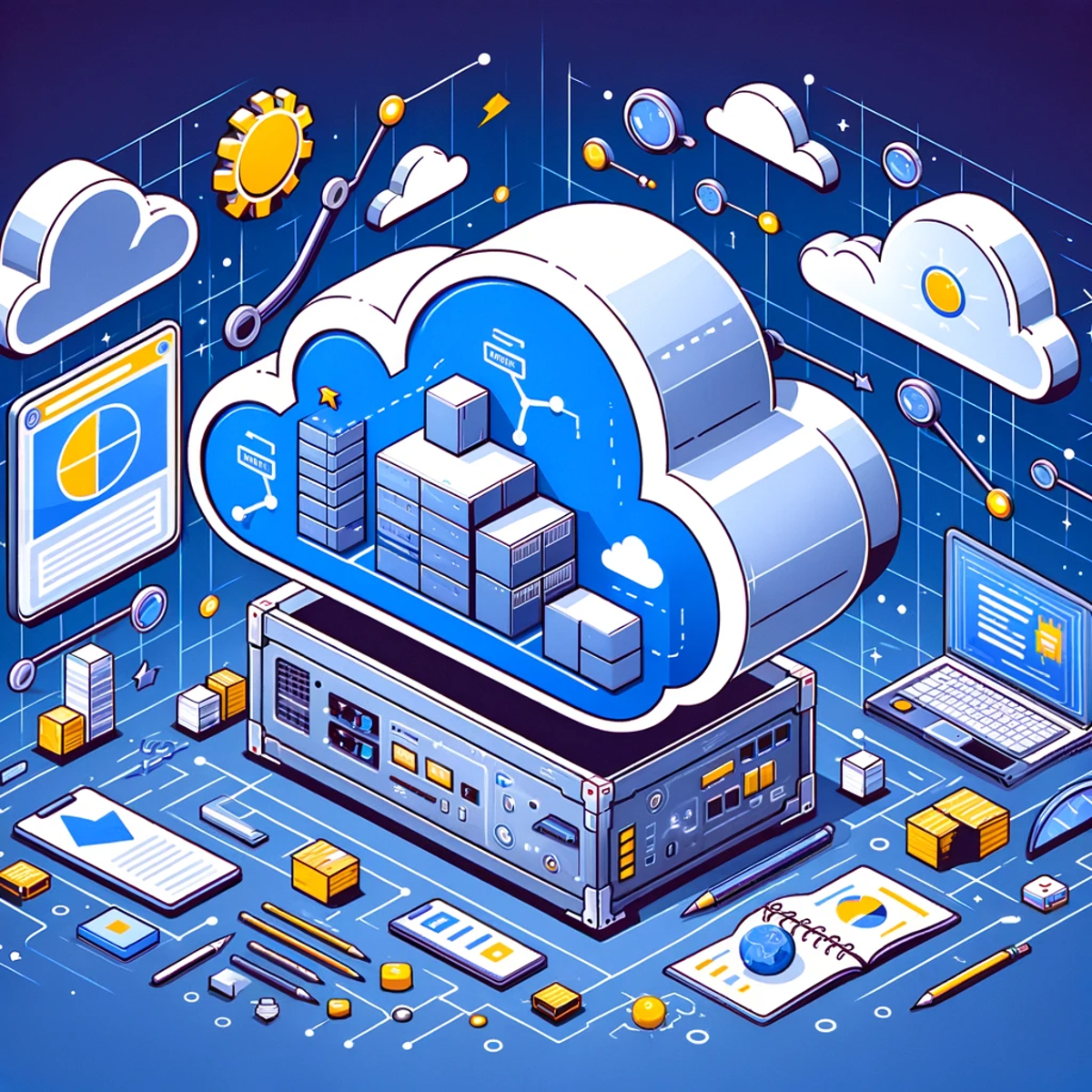
Azure App Service is a powerful and scalable solution for hosting Umbraco applications in the cloud
Cloud Computing: The Sky's the Limit
In today's world, cloud computing isn't just an option.
It's the go-to choice for hosting web solutions, and Umbraco is no exception.
Leveraging the power of cloud computing, especially through services like Microsoft Azure, can elevate your Umbraco projects to new heights.
Let's break down some of Azure key components you need to learn as Umbraco developer:
Azure App Service
It's where you host your Umbraco web app.
Think of it as a secure, comfy home for your site, with the added perks of being super scalable.
Azure SQL Database
Umbraco stores all its data in a database.
Azure SQL is like Fort Knox for your data – fully managed, secure, and high-performing.
Azure Blob Storage
Perfect for keeping all your media files, like images and videos.
It's like a massive digital library that's both secure and easy to access.
💡Quick Tip: If you want to organize your Umbraco assets without chaos - install Dedicated Media Folder package.
Azure Redis Cache
Speed up your site by remembering (caching) the stuff that users often ask for.
Less strain on the database and quicker loading times for users.
Azure Search
It takes the search functionality on your site to the next level.
Imagine being able to find a needle in a haystack but faster.
Azure CDN (Content Delivery Network)
It helps deliver your static content (like CSS, JS, and images) super fast by storing it closer to where your users are.
Application Insights
Keep an eye on how your site's doing.
It's like having a high-tech health monitor for your web app, giving you real-time updates.
Azure DevOps Services
Automates the nitty-gritty of getting your app from development to live.
Think of it as a well-oiled conveyor belt for your code.
Azure Active Directory (AAD)
Manages who gets into your app and what they can do.
It's like having a bouncer for your web application.
Azure Key Vault
Safely stores all your sensitive info, like passwords and keys.
JavaScript, AngularJs and Modern Frameworks: The Power Trio
JavaScript? It's your key to interactive websites.
No fluff, just pure dynamism.
AngularJs takes it up a notch, especially in Umbraco.
It's the backbone of Umbraco's back office, making it responsive and powerful.
AngularJs in Umbraco
Get this: Umbraco's back office is built on AngularJs.
It's all about customizing your Umbraco admin panel to look and do exactly what you need.
You must learn how to craft property editors, dynamic dashboards, sections, custom apps, etc.
Start here:
- Review Umbraco's built-in property editors.
- Creating a custom property editor.
Check out this AngularJs tutorial to learn more about AngularJs.
Frontend Frameworks: Choose Your Fighter
On the front end?
It's a playground. React, Vue.js, Svelte - pick one and make your UI pop.
React's fast, Vue's intuitive, Svelte's sleek.
Mix and match with Umbraco for front-end magic.
In short: Master JavaScript and AngularJs for Umbraco power.
Experiment with modern frameworks for slick UIs.
Keep it lean, keep it smart. 🚀🔧💡
CSS, SAAS, LESS: Your Web Styling Wizards
When it comes to web development, looks matter.
CSS, SAAS, and LESS?
They're your style gurus, your web wardrobe experts.
They transform the bland into the grand, turning basic code into visually stunning websites.
Styling: More Than Just Looks
For a web developer, styling isn't just about making things look good.
It's about creating experiences.
It's the difference between a site that's just there and one that grabs and holds attention.
CSS: The Foundation
CSS is your starting point.
It's like the basic tee - essential and versatile.
But to really stand out, you need more.
SAAS & LESS: The Game Changers
Enter SAAS and LESS.
They take CSS and supercharge it.
Think of SAAS as that statement piece in your wardrobe.
It adds flair and function to your basic CSS, making your styling efficient and your site more responsive.
Action Point: Play with SAAS
Got a site that's looking a bit plain?
Inject some SAAS into it.
It's like accessorizing a basic outfit - suddenly, everything pops!
With SAAS, you can create richer, more dynamic styles and see how it elevates your site's design.
Remember, in web development, styling is as crucial as the code itself.
It's what makes your site memorable.
So, don't just code; style.
Experiment, innovate, and watch as your websites transform from standard to standout. 🎨
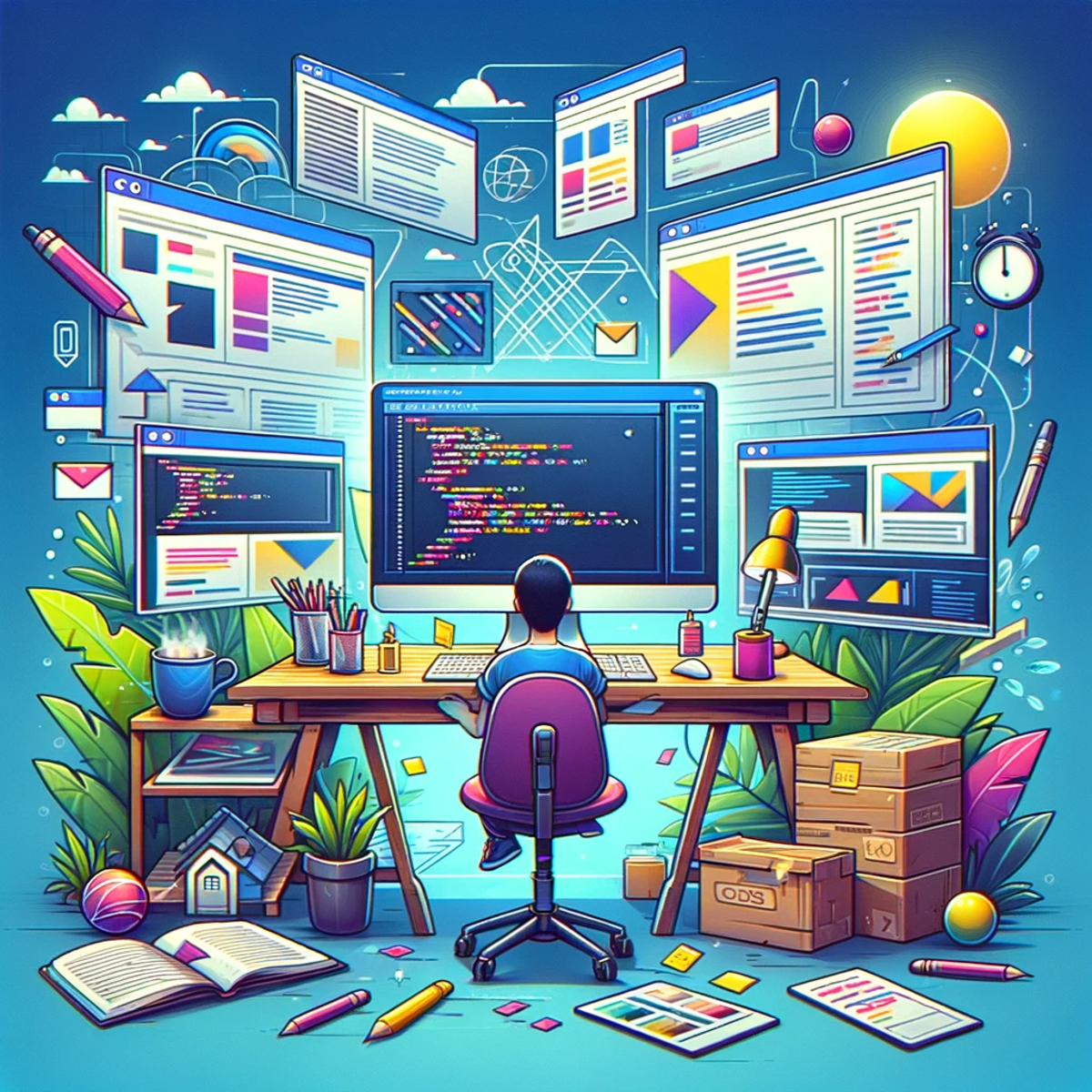
Web Development and Razor Syntax: The Craftsmen
Razor Syntax bridges the gap between the back-end and front-end for Umbraco developers.
This makes your web development process more fluid and intuitive.
Razor example:
Here’s a Razor snippet to display content from Umbraco:
@Umbraco.Content(1234).Name
It displays the name of an Umbraco content item with the ID 1234.
Dive Deeper into Razor
To truly master Razor and elevate your web development skills, explore the official documentation.
It’s packed with resources, examples, and best practices that will help you harness the full potential of Razor Syntax in your Umbraco projects.
Regular Expressions: The Problem Solvers
Jon Franklin once said, "Simplicity carried to the extreme becomes elegance."
This is particularly true for Regular Expressions (Regex).
Simplicity carried to the extreme becomes elegance.
Regex is your secret weapon for text processing.
It slices and dice text to find exactly what you need.
Like a detective with a keen eye for detail, Regex allows you to sift through the chaos of text, identifying, fixing, and extracting precisely what you need with precision and finesse.

Example: Wrapping Text with Regex
Imagine you're working with a Umbraco Rich Text Editor and need to wrap plain text with a <p> tag.
Regex becomes your tool of choice to handle this task elegantly.
Here's an example of how you can achieve this in C#:
public static string WrapPlainTextWithParagraph(this string input)
{
if (!input.IsSet())
return input;
if (input.Contains("<p>"))
return input;
input = $"<p>{input.Trim()}</p>";
input = input.ReplaceNewLines("</p><p>");
return Regex.Replace(input, "\\s+</p><p>+\\s+", "</p><p>", RegexOptions.IgnoreCase);
}
Tip: Check out Expresso for Regular Expressions.
It's a great tool for developers and saves hours of work.
Remember: “With great power comes great responsibility”.
Use regex wisely!
SEO: The Whisperers to Google
SEO isn’t just about keywords and backlinks.
These days are over.
It’s about understanding how Google sees your site and being heard on the vast internet.
SEO Tips:
- Use semantic HTML 5 and descriptive URLs.
- Focus on unique, well-organized, quality content.
Google will thank you and quality leads will make you a ton of money.
Google only loves you when everyone else loves you first.
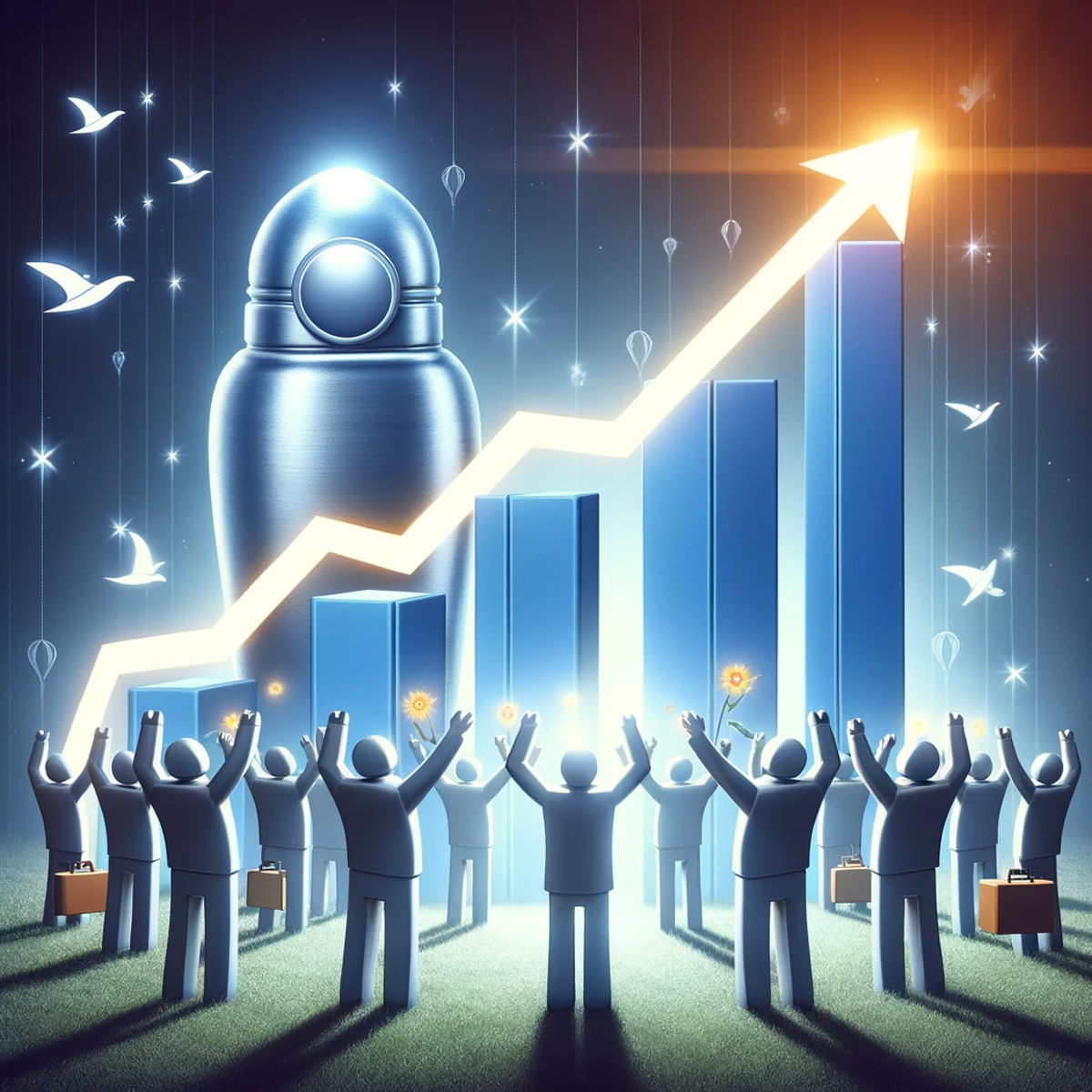
.NET Backend and API Integrations: The Connectors
In the .NET universe, especially with Umbraco, API integrations are essential.
They're like the bridges allowing your applications to communicate, share data, and work together seamlessly.
Real-World API Integrations in Umbraco
Think about a real-life scenario:
You've got an Umbraco site and you need to integrate a third-party payment system or maybe pull in social media feeds.
API integrations make this possible.
They allow your Umbraco site to connect with external services, expanding its capabilities beyond just content management.
Example of API Integration
Let's say you want to display the latest tweets on your Umbraco site.
You'd use the Twitter API to fetch this data.
Here's a simplified example:
public IList<Tweet> GetLatestTweets()
{
// Code to connect to Twitter API and fetch latest tweets
}
This function would communicate with Twitter's API, retrieve the latest tweets, and return them in a format your Umbraco site can display.
Skills for API Integration
To nail API integrations, you need a mix of skills:
- Understanding RESTful Services: Know how web services work, especially REST APIs.
- JSON/XML Proficiency: Be comfortable with data formats like JSON or XML used in API communication.
- Authentication Know-How: Understand OAuth or other authentication methods for secure connections.
- Error Handling: Be prepared to handle potential issues or communication errors gracefully.
API integrations in Umbraco are about making your site not just a standalone entity but a part of a larger, interconnected web ecosystem.
Mastering these integrations means you can expand the functionality of your sites, making them more dynamic and resourceful.

Umbraco works great with external data sources and services
Advanced SQL Queries: The Data Ninjas
The world is one big data problem.
SQL is the language of data.
Knowing how to write advanced queries is like having a ninja skill in your arsenal.
SQL in Action: Finding and Reporting
Consider this scenario.
You need to generate a report on all current versions of articles in your Umbraco CMS that were updated after a specific date.
Here's where your SQL prowess comes into play.
By using a query like the one below, you can efficiently retrieve a detailed list:
declare @now datetime = getdate()
declare @dateFrom datetime = getdate()
declare @days int = 7 -- last 7 days
set @dateFrom = dateadd(day,-@days,@now)
/* List all current versions of documents newer than @dateFrom Trimmed view */
select
umbdocument.nodeId,
umbContentVersion.versionDate,
umbContentVersion.[text],
umbcontentType.alias,
umbracoUser.userEmail,
umbracoUser.[username]
from [umbracoDocument] umbdocument
join [umbracoContent] umbContent on umbdocument.nodeId = umbContent.nodeId
join [cmsContentType] umbcontentType on umbContent.contentTypeId = umbcontentType.nodeId
join [umbracoContentVersion] umbContentVersion on umbContent.nodeId = umbContentVersion.nodeId
join [umbracoUser] umbracoUser on umbContentVersion.userId = umbracoUser.id
where umbContentVersion.[current] = 1
and umbContentVersion.versionDate > @dateFrom
order by umbContentVersion.versionDate desc
This query is a prime example of how SQL filters and organizes data meaningfully, making report generation a breeze.
GDPR Compliance: Deleting Umbraco Users
When it comes to GDPR compliance, SQL can be your ally.
Need to delete Umbraco users permanently for privacy reasons?
A well-crafted SQL query can precisely handle this, ensuring compliance and data integrity.
💡Learn more How to Delete Umbraco User Permanently.
Database Maintenance: Keeping It Clean
As a data ninja, your role extends beyond retrieval and reporting.
Regular database maintenance is crucial.
It includes keeping the database optimized and clean.
This involves periodic checks, updates, and clean-ups to ensure your Umbraco system runs smoothly and efficiently.
In conclusion, SQL is more than just a programming language
It's a fundamental skill for Umbraco developers.
It empowers you to delve into data, make informed decisions, maintain compliance, and ensure the overall health of your Umbraco projects.
Git: The Timekeepers of Your Code
Think of Git as the ultimate time machine in the coding universe.
It’s like having a diary that meticulously records every twist and turn in your development journey.
With Git, every change you make is tracked, giving you the freedom to experiment and evolve your code without ever losing your way.
Git Flow: The Roadmap for Efficient Development
Diving deeper into Git, there's a concept called 'Git Flow' that's pretty crucial.
It's like having a GPS for your coding journey.
Git Flow lays out a clear workflow, helping you organize how you build, test, and release your code.
Want to learn more?
Check out this guide on comparing workflows with Git Flow.
It breaks down the process and shows you why it's essential for keeping your projects organized and efficient.
Why Git Flow is Essential
- Organized Coding: Git Flow keeps your work structured - no more messy, confusing code.
- Easier Collaboration: Working with a team? Git Flow makes it smooth to combine everyone's work without stepping on each other's toes.
- Safe Experimentation: Want to try something new? Do it without messing up your main project, thanks to feature branches.
When using git, it's recommended to:
Develop a Feature
Start a new feature branch for each feature you're working on.
It's like giving each idea its own workspace.
Merge Regularly
Once a feature is ready, merge it back into your main branch.
Think of it as adding completed puzzle pieces to the big picture.
Release and Tag
When your project hits a milestone, release a version and tag it.
It’s like putting a bookmark in your diary to mark a special event.
Maintenance
If you find bugs, work on them in a separate branch and merge the fixes back.
It’s like doing repairs without disrupting the whole house.
Remember the Git wisdom: "Commit often, push regularly."
Your future self, and maybe your team, will definitely thank you.
With Git and Git Flow, you’re not just coding.
You’re crafting a well-organized, collaborative, and history-rich masterpiece. 🌟

Git feature branches. Source: https://www.atlassian.com/
Soft Skills: The Human Element in Coding
Coding skills are paramount in development, especially in complex fields like Umbraco.
But there's a human factor equally crucial for success - soft skills.
They're the bridge between technical excellence and real-world impact.
Let's explore how you can actively develop these skills with practical tips:
Communication - the human connection - is the key to personal and career success.
The Art of Communication
Quick Tip: Practice active listening in your next team meeting or client call.
Focus on understanding the speaker's perspective before responding.
When explaining technical concepts, use analogies or simple terms.
Think of it as translating code into a story.
Understanding Client Needs
Quick Tip: Next time you receive a project brief, schedule a follow-up meeting to ask clarifying questions.
Show empathy by acknowledging their concerns and offering reassurances backed by your technical knowledge.
It's like being a tech detective, piecing together clues to solve their puzzle.
Problem-Solving with Emotional Intelligence
Quick Tip: In stressful situations, practice mindfulness or take a short break to regain composure.
Encourage open dialogue within your team to share concerns and brainstorm solutions.
Act as the team's emotional thermostat, helping regulate the collective mood and stress levels.
Building Connections
Quick Tip: Network with other developers and professionals in the tech community.
Attend webinars, join forums, or participate in local meetups (virtual or in-person).
Sharing experiences and learning from others can significantly enhance your interpersonal skills.
Developing Empathy
Quick Tip: View challenges from the perspectives of others, clients, or colleagues.
For a week, make a conscious effort to consider how your actions and decisions might affect others in your team or project.
Paul J. Meyer's words, "Communication - the human connection - is the key to personal and career success," ring true in tech as much as in any other field.
By actively working on these soft skills, you become a better developer, a valued team member, and a trusted advisor to your clients.
Remember, human elements like empathy, understanding, and communication are your superpowers in the digital world.
In Conclusion
Our exploration of the essential skills for Umbraco developers is just the tip of the iceberg.
The tech world is ever-evolving, and so is Umbraco.
Staying up-to-date and continuously honing developer skills is not just beneficial.
It's essential.
But don't worry.
Umbraco isn't just a platform - it's a community rich with resources and expertise.
🛠️ Got Umbraco Puzzles to solve?
We're here to help.
From expert SEO audits to deploying cutting-edge features to the cloud, our experts are just a click away.
Reach out, and let’s tackle these challenges head-on! 👨💻👩💻