Jump to Section
Why Search Text Across SQL Server Tables?#
In a complex database, pinpointing where specific data resides can be tricky.
For example, you might need to:
- Find all instances of a customer’s name across different tables.
- Audit records that reference a specific keyword.
- Search unknown or undocumented schemas.
Query to search text in a SQL Server database #
Here’s the heart of our solution - a T-SQL script that scans text columns for a specific string.
The Code:
Key Points
- Adjust @TargetString for your search term.
- Modify the DATA_TYPE filter to match your database's column types.
- Test in a non-production environment to evaluate performance.
How It Works#
This script uses three core concepts:
1. Cursors
Iterates through all columns that match the specified data types. While efficient for this use case, cursors can be slower than set-based operations in large datasets.
2. Dynamic SQL
Dynamically builds and executes queries for each column. To avoid SQL injection risks, use parameters (e.g., sp_executesql with parameters) in production scenarios.
3. Data Types
Filter columns are based on their types (e.g., varchar, nvarchar) and are only used to target text-based data.
Benefits and Limitations#
Advantages
- Comprehensive Search: Scans all text-based columns across all tables.
- Flexible: Easily adaptable for different schemas, tables, or data types.
- Dynamic: Handles unknown database structures without requiring schema documentation.
Drawbacks
- Performance Overhead: May be slow on large databases. Consider running during off-peak hours.
- Cursor Inefficiency: Cursors can impact performance more than set-based operations.
- SQL Injection Risk: Ensure proper sanitization to secure dynamic queries.
Example Output#
Running the script yields results similar to these:
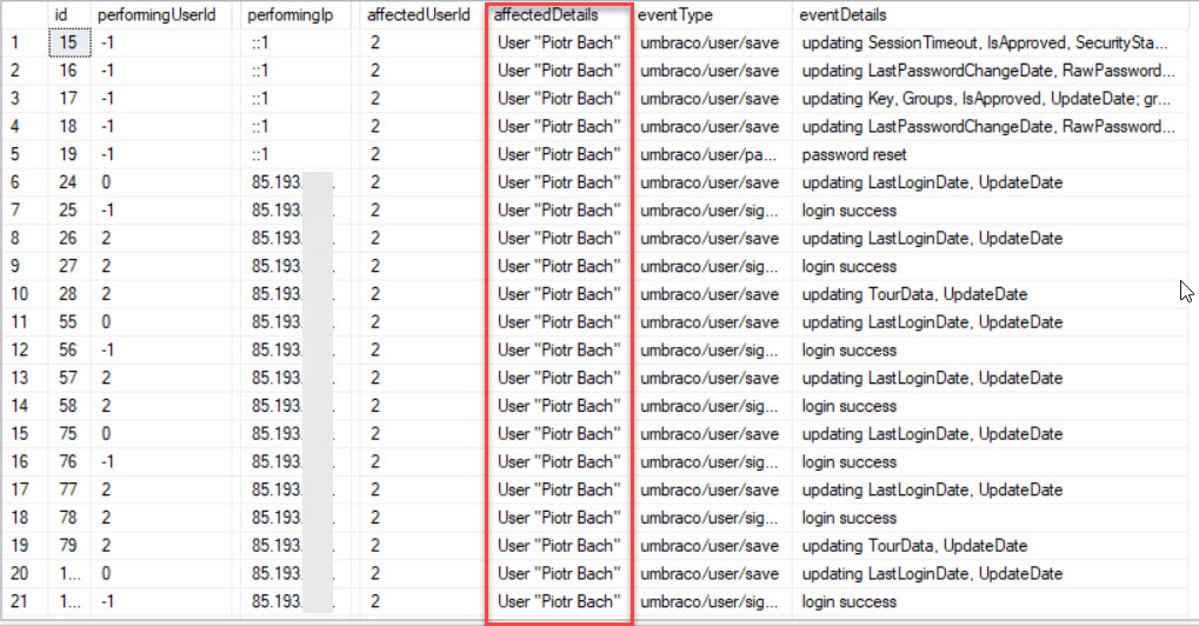
This output confirms where the search term appears and highlights the affected rows.
Best Practices#
- Test Before Production: Always run the script in a staging environment first.
- Optimize Data Types: Narrow the search to only necessary columns for better performance.
- Monitor Impact: Use SQL Profiler or other tools to gauge the performance impact on your database.
Next Steps#
💡 Try It Now! Copy and customize the script for your database.
🚀 Have an Idea for Improvement? It's all about collaboration!
Visit the GitHub repository to share your suggestions or contribute directly.
🌐 Learn More
Visit our blog for practical SQL tips.