Table of contents
Optimizing your website's scripts not only speeds up the loading time but also enhances the user experience.
In this guide, we'll explore ASP.NET's in-built script optimization tools, detailing the steps to bundle and minify your website scripts effectively.
Setting Up: Installing the Required Package
Before diving into the main steps, ensure you have Microsoft.AspNet.Web.Optimization package in your project. If not, swiftly add it using NuGet:
Install-Package Microsoft.AspNet.Web.Optimization
Crafting the BundleConfig
Navigate to the App_Start folder (create one if missing) and set up a BundleConfig.cs file.
This file acts as the control center for your scripts and their bundles.
public class BundleConfig
{
public static void RegisterBundles(BundleCollection bundles)
{
//create bundles and add them to BundleCollection
}
}
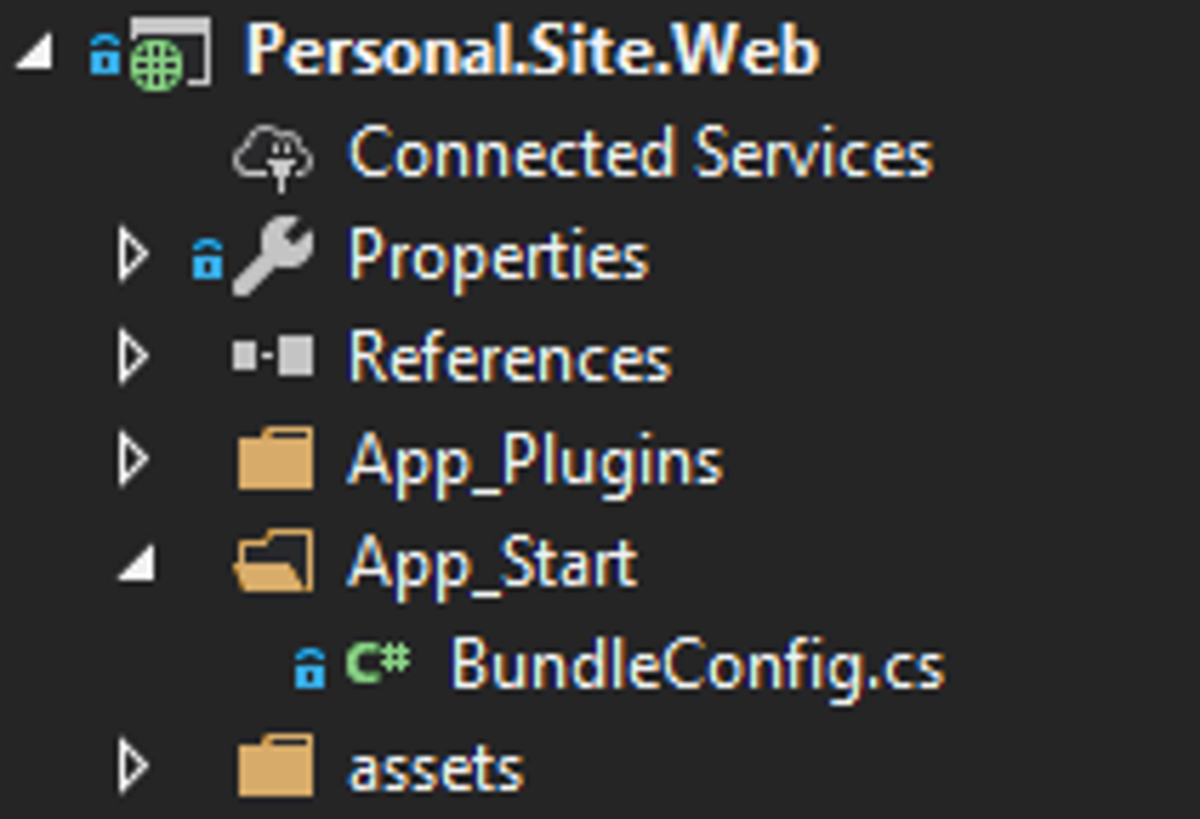
BundleConfig file in ASP .NET project structure
Designing Effective Bundles
Group your scripts into relevant bundles.
For example, you might need different bundles for home and blog pages.
Here's an illustrative example showcasing how you can create script and style bundles:
public class BundleConfig
{
public static void RegisterBundles(BundleCollection bundles)
{
ScriptBundle scriptBundle = new ScriptBundle("~/bundles/master/js");
scriptBundle
.Include("~/assets/js/jquery.js")
.Include("~/assets/js/plugins.js")
.Include("~/assets/js/functions.js");
bundles.Add(scriptBundle);
StyleBundle styleBundle = new StyleBundle("~/bundles/master/css");
styleBundle
.Include("~/assets/css/plugins.css")
.Include("~/assets/css/style.css")
.Include("~/assets/css/custom.css");
bundles.Add(styleBundle);
BundleTable.EnableOptimizations = true;
}
}
This code is an example of how ASP.NET (specifically, ASP.NET MVC) can be used to bundle and minify multiple CSS and JavaScript files.
Bundling technique helps improve the performance of web applications by reducing the number of requests to the server and minimizing the size of the static files.
Ensuring Optimization
Ensure that the debugging mode is turned off to allow bundling and minification:
<system.web>
<compilation debug="false" />
</system.web>
BundleTable.EnableOptimizations = true
Initializing the Bundling Engine
In the Global.asax.cs file, invoke the bundling engine at application start:
protected void Application_Start(Object sender, EventArgs e)
{
BundleConfig.RegisterBundle(BundleTable.Bundles);
}
Implementing Bundles in Razor Views
For utilizing bundles in your Razor views:
- Add the System.Web.Optimization namespace to the ~/View/Web.config file.
- Use the @Scripts and @Styles helpers in your Razor View:
<add namespace="System.Web.Optimization"/>
@using System.Web.Optimization;
<!DOCTYPE html>
<html lang="en-US">
<head>
@Styles.Render("~/bundles/master/css")
</head>
<body>
@RenderBody()
@Scripts.Render("~/bundles/master/js")
</body>
</html>
Overcoming Browser Cache Hurdles
Have you ever made changes only for someone to report they "don't see them"?
Browser caching is often the culprit.
There is a good old trick to make sure the browser will work with the appropriate versions of scripts/bundles.
It’s straightforward to force the browser cache to use the latest CSS/JS bundles by appending the query string parameter at the end of each bundle.
<link href="/bundles/master/css" rel="stylesheet"/>
Each bundle should have a unique URL after each build:
<link href="/bundles/master/css?v=rJwD8GQBqCoQ6sc6KrlHuWYflkjwRDAm2RK3fPv7aOk1" rel="stylesheet"/>
After googling here and there, I found a great C# extension on StackOverflow.
All credit goes to author Ranjeet patil (thanks)!
internal static class BundleExtensions
{
public static Bundle WithLastModifiedToken(this Bundle sb)
{
sb.Transforms.Add(new LastModifiedBundleTransform());
return sb;
}
public class LastModifiedBundleTransform : IBundleTransform
{
public void Process(BundleContext context, BundleResponse response)
{
foreach (var file in response.Files)
{
var lastWrite = File.GetLastWriteTime(HostingEnvironment.MapPath(file.IncludedVirtualPath)).Ticks.ToString();
file.IncludedVirtualPath = string.Concat(file.IncludedVirtualPath, "?v=", lastWrite);
}
}
}
}
Using this clean and awesome CSharp extension method – we get an elegant and effective code inside BundleConfig.cs:
public class BundleConfig
{
public static void RegisterBundles(BundleCollection bundles)
{
ScriptBundle scriptBundle = new ScriptBundle("~/bundles/master/js");
scriptBundle
.Include("~/assets/js/jquery.js")
.Include("~/assets/js/plugins.js")
.Include("~/assets/js/functions.js");
bundles.Add(scriptBundle.WithLastModifiedToken());
StyleBundle styleBundle = new StyleBundle("~/bundles/master/css");
styleBundle
.Include("~/assets/css/plugins.css")
.Include("~/assets/css/style.css")
.Include("~/assets/css/custom.css");
bundles.Add(styleBundle.WithLastModifiedToken());
BundleTable.EnableOptimizations = true;
}
}
Now, after each build – you will get a unique extra parameter in your script URL:
<link href="/bundles/master/css?v=rJwD8GQBqCoQ6sc6KrlHuWYflkjwRDAm2RK3fPv7aOk1" rel="stylesheet"/>
This technique should resolve browser caching problems and make end-users happy.
Celebrating Your Optimized Output
With the steps above, your bundles are not only optimized but also effectively managed.
Here's how your output should appear:
<link href="/bundles/master/css?v=unique-version" rel="stylesheet"/>
<script src="/bundles/master/js?v=unique-version"></script>
Conclusion
Enhancing website performance is crucial for user satisfaction and SEO.
Bundling and minification in ASP.NET offer an intuitive way to achieve this.
With the steps outlined above, your website should be swift and efficient, leading to a seamless user experience.
Interested in more ASP.NET & ASP.NET Core tips and tricks?
Dive deeper into our blog, or contact us for personalized assistance!