Dynamic Record Counting Script
Understanding data spread across tables is essential for analytics and maintenance in large databases.
The following T-SQL script counts records in each table of a specified schema and sorts the results by record count in descending order.
Example Output
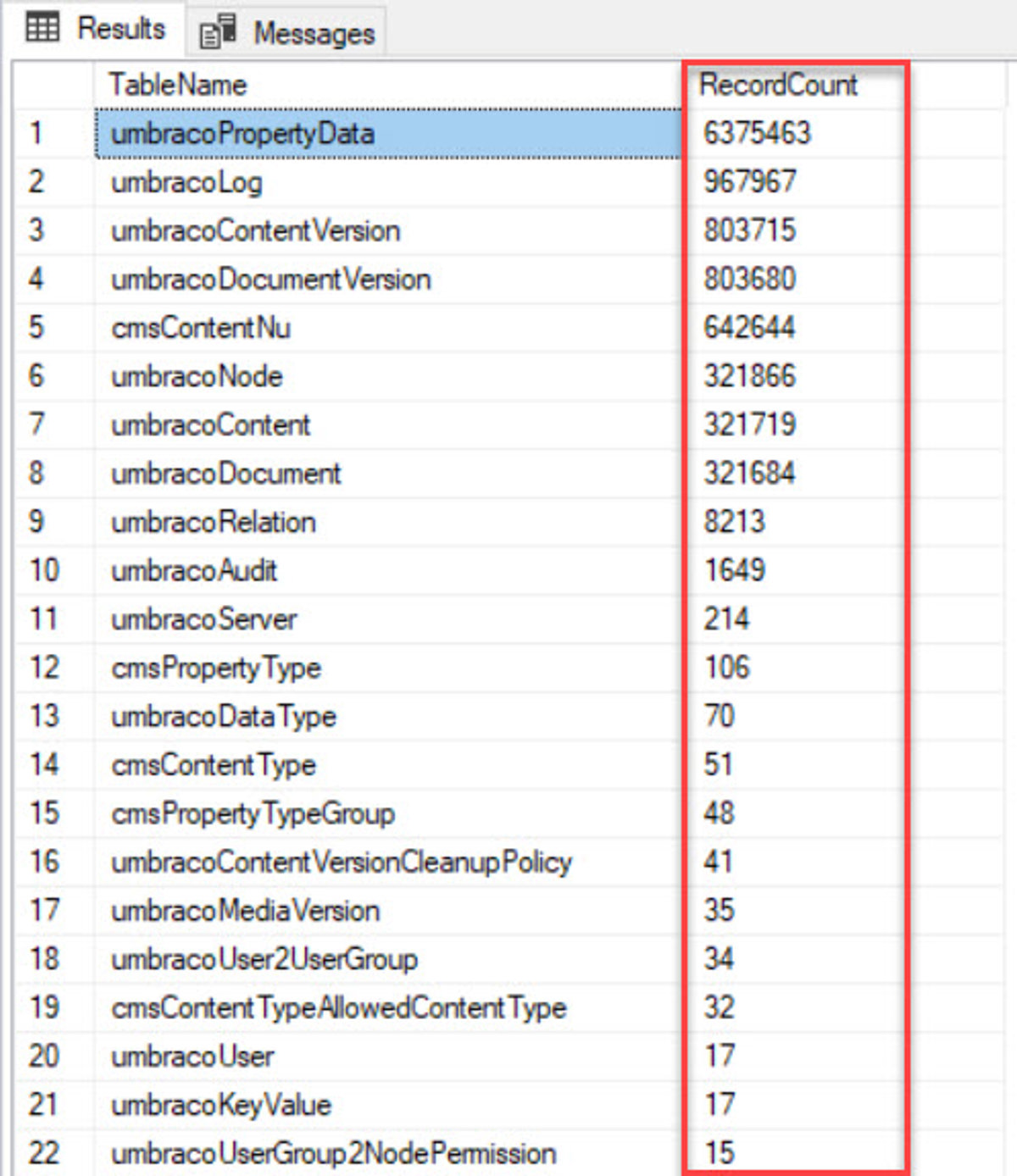
Key Points
- Cursors: Loop through tables in the schema and process them individually.
- Dynamic SQL: Build and execute SQL queries for each table dynamically.
- Temporary Table: Store and sort record counts for efficient presentation.
Concluding Insights#
This script is a straightforward tool for database analytics, providing quick insights into table record counts.
Modify the @SchemaName variable to fit your needs.
The code is available on GitHub
Be cautious when using it on a production database, as performance may be impacted, especially with large tables or high concurrency.