Understanding the Problem
Due to deserialization errors, the default LogViewer in Umbraco may fail to parse JSON-formatted log files.
This issue is well-documented, as seen in our blog post Fixing the Reader's MaxDepth of 64 Has Been Exceeded in Umbraco.
We submitted a pull request to the Umbraco core repository to address this.
During discussions, Bjarke Berg suggested an alternative: replacing the default LogViewer with a custom implementation.
Here, we’ll show you how to implement this solution.
Steps to Replace LogViewer in Umbraco v13
To replace the default LogViewer, you’ll need to:
-
Create a Composer
-
Implement a Custom LogViewer
-
Register the Custom LogViewer
Let’s break down each step in detail:
Step 1: Creating a Composer
A composer in Umbraco allows you to configure the application during startup.
The CustomLogViewerComposer class is a simple implementation of IComposer, which adds your custom LogViewer to the builder:
This composer ensures that your custom LogViewer replaces the default implementation during application startup.
Step 2: Implementing a Custom LogViewer
The custom LogViewer class extends SerilogLogViewerSourceBase and provides a more robust implementation.
Here’s a snippet of the UmbracoCustomLogViewer:
Key features include:
-
Custom JSON Serializer: Adjusts MaxDepth and handles missing or null values gracefully.
-
Error Handling: Implements detailed logging for JSON parsing errors to avoid crashes.
Optimizing the UmbracoCustomLogViewer class is beyond the scope of this article. We decided to keep the original Umbraco code for better readability and future investigative purposes. Note the correct error handling.
Step 3: Registering the Custom LogViewer
Finally, register your custom LogViewer using an extension method on IUmbracoBuilder:
This method ensures that your custom LogViewer replaces the default implementation.
Step 4: Testing the LogViewer Implementation#
After implementing and registering the custom LogViewer, verifying its functionality is essential.
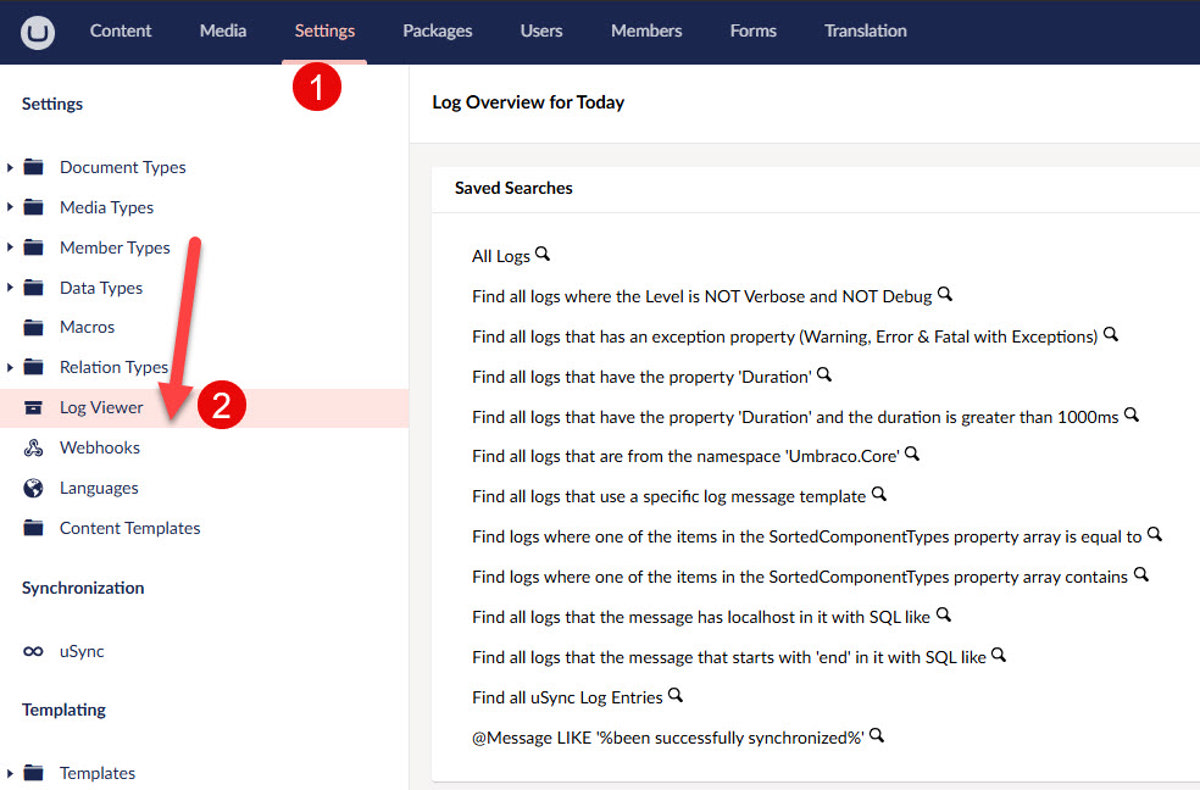
Navigating to Umbraco LogViewer in Backoffice
Navigate to the LogViewer in the Umbraco back office:
1. Go to /umbraco#/settings/logViewer/overview.
2. Perform smoke tests to ensure proper functionality:
- Display all error logs.
- Navigate through multiple pages of logs to review older entries.
- Confirm that no errors occur while loading logs.
These steps will validate that the custom LogViewer handles logs correctly and resolves the issues encountered with the default implementation.