Jump to Section
Removing an Examine index in Umbraco can improve performance in various ways, especially on large, content-heavy websites.
In the previous article How to Disable Examine External Indexing in Umbraco, I walked through the process of disabling the Examine External Index
Now, I would like to demonstrate a more flexible approach allowing you to disable any Examine index.
The solution below has been tested on two versions of Umbraco 11 and 13, but it should also work on subsequent and newer ones.
Reviewing Active Umbraco Indexes in Umbraco Backoffice#
When you go to the 'Settings' section and select the 'Examine Management' tab, you will see all active Examine indexes:
- DeliveryApiContentIndex
- ExternalIndex
- InternalIndex
- MembersIndex
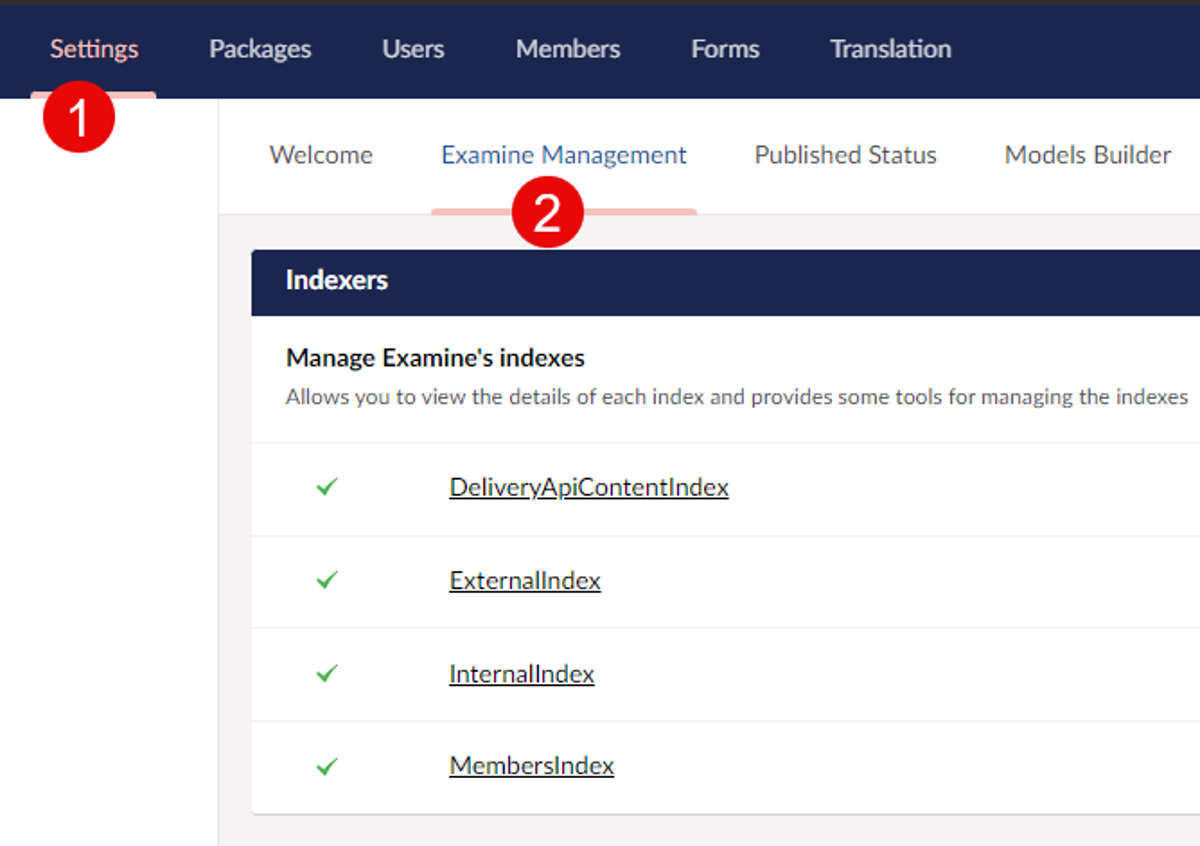
Active Examine indexes in Umbraco Settings view
This means that all four indexes are active.
Understanding Registering Examine Indexes in Runtime#
In Umbraco, indexes are typically registered through the ServicesCollectionExtensions.cs file using the AddExamineLuceneIndex<TIndex, TDirectoryFactory>() method.
Here's a simplified look at how this registration process works:
In the above code, the AddExamineLuceneIndex method registers a new Examine index in Umbraco using dependency injection.
It defines various configurations for the index, including the analyzer, validator, and directory factory, which are crucial components in the Lucene indexing system.
Removing Examine Index by Name#
Now, to remove an Examine index by its name, you can use the following extension method:
As you can see - RemoveExamineIndex method takes advantage of C# reflection to find the correct index.
The method inspects all registered IIndex services, finds the ones matching the specified indexName, and removes them from the services collection.
Removing Umbraco ExternalIndex and DeliveryApiContentIndex#
Let's say you want to remove two indexes: ExternalIndex and DeliveryApiContentIndex.
You just need to register a Composer and call the RemoveExamineIndex method twice with the appropriate index name as below:
This will cleanly remove the ExternalIndex and DeliveryApiContentIndex during the application startup process, improving your site's performance by eliminating unnecessary indexing tasks.
Notice that the index names are defined in Umbraco constants:
Validating Active Umbraco Indexes Again#
Now, when you restart Umbraco, you will see in the system settings that only two indexes are active, as show in the screenshot below:
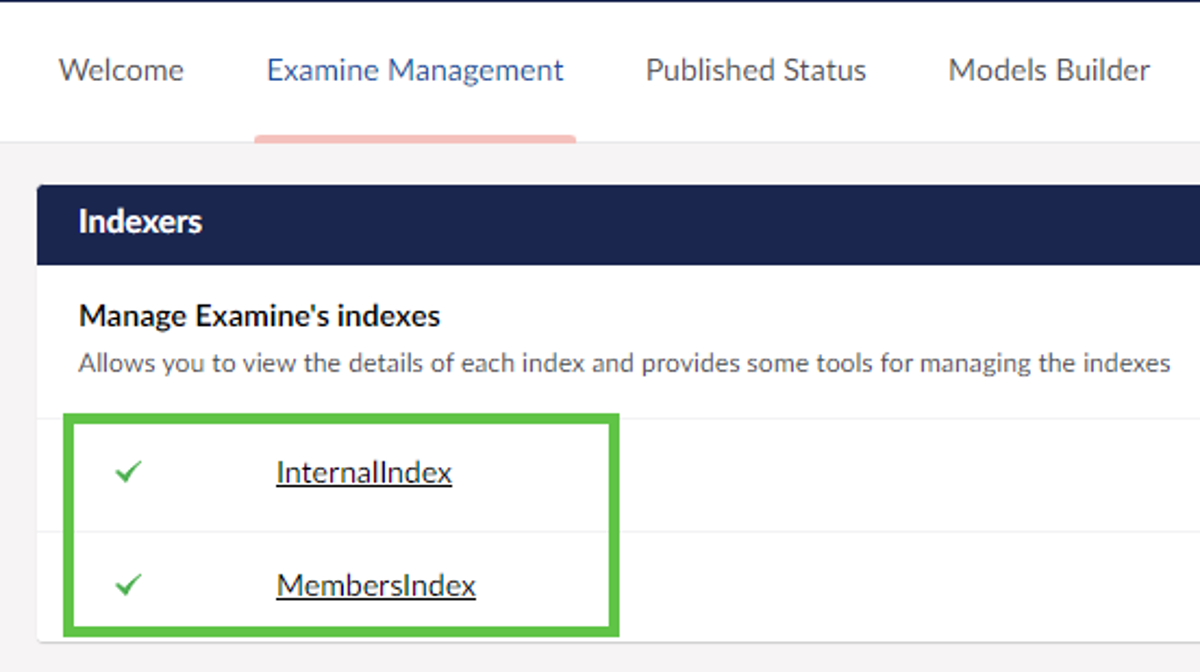
Active Examine indexes in Umbraco Settings view after index removal
Benefits of Removing Examine Indexes#
When working with Umbraco, every active Examine index adds overhead to your system.
These indexes continually track changes to your content, ensuring the search functionality remains up-to-date.
However, there are scenarios where this indexing might be unnecessary or even detrimental:
Improved Performance
Disabling unnecessary indexes can lead to faster content publishing and reduced overall processing load.
Each index requires system resources to maintain its state, especially during large data imports or high-traffic scenarios.
Removing them reduces the indexing time, which improves cold start-ups and system responsiveness.
Simpler Search Management
By removing unused indexes, you streamline your search infrastructure.
This can simplify maintaining and troubleshooting search-related issues, as you're focusing only on what's necessary for your use case.
Optimized Content Queries
In some cases, custom searches (such as those that don’t rely on Umbraco's default indexes) are more efficient for specific types of content.
Removing irrelevant indexes makes it easier to implement custom solutions without conflicts.
Pain Points and Considerations#
When removing Examine indexes in Umbraco, there are a few potential challenges to remember.
Breaking Features that Depend on the Index
If your website has a custom search feature that relies on the ExternalIndex, disabling this index could break search functionality.
Imagine users suddenly being unable to search for products on an e-commerce site because the index powering the search no longer exists.
Issues with Third-Party Plugins
A third-party SEO plugin that relies on ExternalIndex to track and analyze content performance could stop functioning correctly.
This could lead to a drop in your site’s SEO performance without you even realizing it.
Testing and Validation Overload
Disabling multiple indexes across a large, complex site can result in unforeseen issues that require extensive testing to identify.
Remember: Removing the InternalIndex will probably kill your Umbraco website immediately.
Conclusion#
In this tutorial, you learned how to disable any Examine index.
Removing unnecessary Examine indexes in Umbraco can be a powerful optimization tool, especially on larger websites or those with highly specialized search needs.
This technique can significantly improve your site's speed, reduce resource usage, and simplify search management.
However, be mindful of the potential side effects and thoroughly test before going live.
You will find other optimization tips here: How to speed up Umbraco CMS performance.
What's Next?
Explore our blog for more insights, and feel free to reach out for any queries or discussions related to Umbraco development.